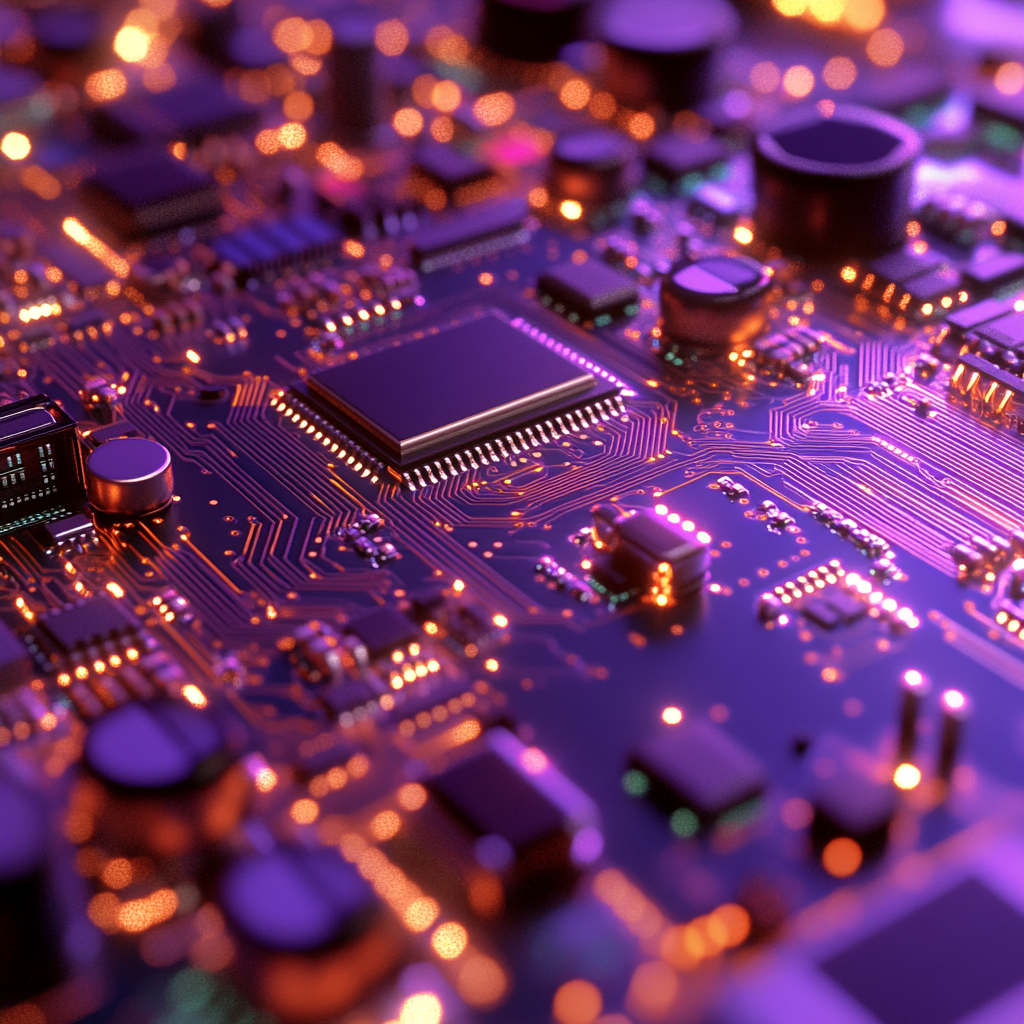
At Sequence, we've developed two libraries that enable secure signing of EVM messages and transactions using cloud-based Key Management Services (KMS). We've built adapters for both AWS KMS and Google Cloud KMS that are fully compatible with ethers.js v6 and any Web3 library supporting ethers v6 signers. Internally, these signers leverage the AWS/GCP clients to interact with their respective cloud services.
With these adapters, you can easily instantiate an AwsKmsSigner
or GoogleKmsSigner
, both extending AbstractSigner
from ethers.js. Simply provide the necessary configuration variables from AWS or GCP, and you can use them directly as ethers v6 signers.
For example, when integrated with Sequence's SDK, these signers can serve as EOA owners for Sequence smart wallets.
We developed these libraries primarily to provide a secure, seamless way to use backend wallets for Sidekick. While I won't elaborate on Sidekick here, it's essentially an open-source backend designed to simplify Web3 interactions for games and apps by offering built-in features like daily rewards, webhook management, and more.
The core idea was to create a backend wallet capable of performing on-chain operations while maintaining high security, made possible through KMS-based signing. This need for a secure, flexible backend wallet drove the development of these libraries.
AWS KMS Signer Implementation
The AwsKmsSigner
class in signer.ts
extends ethers.AbstractSigner
and integrates with AWS KMS to perform signing operations securely.
Let's examine each method:
Class Variables and Constructor
private address?: Promise<string>;
private pubkey?: string;
private readonly client: () => KMSClient;
private readonly keyId: string;
private readonly region: string;
constructor(region: string, keyId: string, provider?: ethers.Provider) {
super(provider);
this.client = () => new KMSClient({ region });
this.keyId = keyId;
this.region = region;
}
address
will store the signer's Ethereum addresspubkey
stores the public key retrieved from AWS KMS- The constructor instantiates the
KMSClient
with the provided region and stores bothkeyId
andregion
for later use
Getting the Signer Address and Signing Messages
getAddress(): Promise<string> {
if (!this.address) {
this.address = this.getPubkey().then(ethers.computeAddress)
}
return this.address
}
signMessage(message: string | ethers.BytesLike): Promise<string> {
return this.signDigest(ethers.hashMessage(message));
}
getAddress()
checks for a cached address value, otherwise it callsgetPubKey()
to fetch itsignMessage()
simply callssignDigest()
with the hashed message
Signing Transactions and Typed Data
async signTransaction(
tx: Deferrable<ethers.TransactionRequest>,
): Promise<string> {
const resolved = await ethers.resolveProperties(tx);
if (resolved.from !== undefined) {
const address = await this.getAddress();
if (resolved.from !== address) {
throw new Error(
`from address is ${resolved.from}, expected ${address}`,
);
}
}
const signature = await this.signDigest(
ethers.keccak256(serialize(tx as UnsignedTransaction)),
);
return serialize(tx as UnsignedTransaction, signature);
}
async signTypedData(
domain: ethers.TypedDataDomain,
types: Record<string, Array<ethers.TypedDataField>>,
value: Record<string, unknown>,
): Promise<string> {
const resolved = await ethers.TypedDataEncoder.resolveNames(
domain,
types,
value,
async (name) => {
if (!this.provider) {
throw new Error(`unable to resolve ens name ${name}: no provider`);
}
const resolved = await this.provider.resolveName(name);
if (!resolved) {
throw new Error(`unable to resolve ens name ${name}`);
}
return resolved;
},
);
return this.signDigest(
ethers.TypedDataEncoder.hash(resolved.domain, types, resolved.value),
);
}
- Both methods perform basic validation before calling
signDigest()
with the appropriate hash
Connecting a Provider
connect(provider: ethers.Provider): ethers.Signer {
return new AwsKmsSigner(this.region, this.keyId, provider);
}
- This method creates a new
AwsKmsSigner
instance with the provided provider, maintaining compatibility with the ethers signer interface
Fetching the Public Key
async getPublicKey(): Promise<string> {
if (!this.pubkey) {
const { PublicKey } = await this.client().send(new GetPublicKeyCommand({ KeyId: this.keyId }));
this.pubkey = Buffer.from(PublicKey!).toString("hex");
}
return this.pubkey;
}
- Uses AWS SDK's
GetPublicKeyCommand
to retrieve the public key from KMS - Converts the binary key data to a hexadecimal string
- Caches the result to avoid redundant API calls
Signing a Digest
private async signDigest(digest: ethers.BytesLike): Promise<string> {
const command = new SignCommand({
KeyId: this.keyId,
Message: getBytes(digest),
MessageType: "DIGEST",
SigningAlgorithm: "ECDSA_SHA_256",
});
const response = await this.client().send(command);
const signatureHex = response.Signature;
if (!(signatureHex instanceof Uint8Array)) {
throw new Error(
`signature is ${typeof signatureHex}, expected Uint8Array`,
);
}
const signature = AsnConvert.parse(
Buffer.from(signatureHex),
ECDSASigValue,
);
let s = toBigInt(new Uint8Array(signature.s));
s = s > secp256k1N / BigInt(2) ? secp256k1N - s : s;
const recoverAddress = recoverAddressFn(digest, {
r: toBeHex(toBigInt(new Uint8Array(signature.r)), 32),
s: toBeHex(s, 32),
v: 0x1b,
});
const address = await this.getAddress();
return Signature.from({
r: toBeHex(toBigInt(new Uint8Array(signature.r)), 32),
s: toBeHex(s, 32),
v: recoverAddress.toLowerCase() !== address.toLowerCase() ? 0x1c : 0x1b,
}).serialized;
}
Creates a SignCommand
with the digest and ECDSA_SHA_256 algorithm, then sends it to the AWS KMS client
- Validates that the response signature is a
Uint8Array
- Parses the ASN.1-encoded signature to extract the r and s values
- Normalizes the s value according to EIP-2
- Determines the correct recovery value (v) by comparing the recovered address with the signer's address
- Returns the serialized signature
Google Cloud KMS Signer Implementation
The GCP KMS signer follows similar logic but with differences in configuration and handling of public keys and signatures.
private address?: Promise<string>
private pubkey?: Promise<string>
private readonly client: () => KeyManagementServiceClient
private readonly identifier: string
constructor(
private readonly path: GoogleKmsKey,
client = new KeyManagementServiceClient(),
provider?: ethers.Provider,
) {
super(provider)
this.client = () => client
this.identifier = this.client().cryptoKeyVersionPath(
this.path.project,
this.path.location,
this.path.keyRing,
this.path.cryptoKey,
this.path.cryptoKeyVersion
)
}
- The constructor takes a
GoogleKmsKey
object containing the necessary path components - It initializes the
KeyManagementServiceClient
and constructs the key identifier string
The main difference lies in the getPubkey()
method, which handles the different format returned by GCP KMS:
private async getPubkey(): Promise<string> {
if (!this.pubkey) {
const [pubkey] = await this.client().getPublicKey({ name: this.identifier })
if (pubkey.algorithm !== 'EC_SIGN_SECP256K1_SHA256') {
throw new Error(`algorithm is ${pubkey.algorithm}, expected EC_SIGN_SECP256K1_SHA256`)
}
if (!pubkey.pem) {
throw new Error('missing public key pem')
}
const PREFIX = '-----BEGIN PUBLIC KEY-----'
const SUFFIX = '-----END PUBLIC KEY-----'
const pemContent = pubkey.pem
.replace(PREFIX, '')
.replace(SUFFIX, '')
.replace(/\\s/g, '')
const derBuffer = Buffer.from(pemContent, 'base64')
const publicKeyInfo = AsnConvert.parse(derBuffer, SubjectPublicKeyInfo)
const publicKeyBytes = new Uint8Array(publicKeyInfo.subjectPublicKey)
const keyBytes = publicKeyBytes[0] === 0x04 ? publicKeyBytes.slice(1) : publicKeyBytes
return `0x${Buffer.from(keyBytes).toString('hex')}`
}
return this.pubkey
}
- Retrieves the public key from GCP KMS and verifies it uses the correct algorithm
- Processes the PEM-formatted key by:
- Removing the PEM header, footer, and whitespace
- Decoding from base64 to binary DER format
- Parsing the ASN.1 structure using
SubjectPublicKeyInfo
from the@peculiar/asn1-x509
library - Extracting the raw key bytes and removing the 0x04 prefix if present (which indicates uncompressed EC point format)
- Converting to a hex string with the '0x' prefix required for Ethereum
The result is an Ethereum-compatible public key that can be used with any Ethereum transaction or message signing operation.
Feel free to explore the implementation and use these libraries in your projects. If you have any questions or feedback, don't hesitate to reach out!
NPM
@0xsequence/aws-kms-signer: https://www.npmjs.com/package/@0xsequence/aws-kms-signer @0xsequence/google-kms-signer: https://www.npmjs.com/package/@0xsequence/google-kms-signer
Github
@0xsequence/aws-kms-signer: https://github.com/0xsequence/aws-kms-signer @0xsequence/google-kms-signer: https://github.com/0xsequence/google-kms-signer
If you’re curious about how we implemented this in Sidekick, have a look, it’s open source ! 👇
https://github.com/0xsequence/sequence-sidekick/blob/master/src/utils/wallet.ts